Ever wondered how your favorite apps handle multiple tasks at once, like loading data or updating content in the background? It’s all thanks to asynchronous programming!
As the world of Android development continues to evolve, mastering asynchronous programming becomes crucial for building responsive and efficient applications. In this blog post, we will explore various asynchronous programming techniques in Kotlin, providing insights into their advantages and challenges
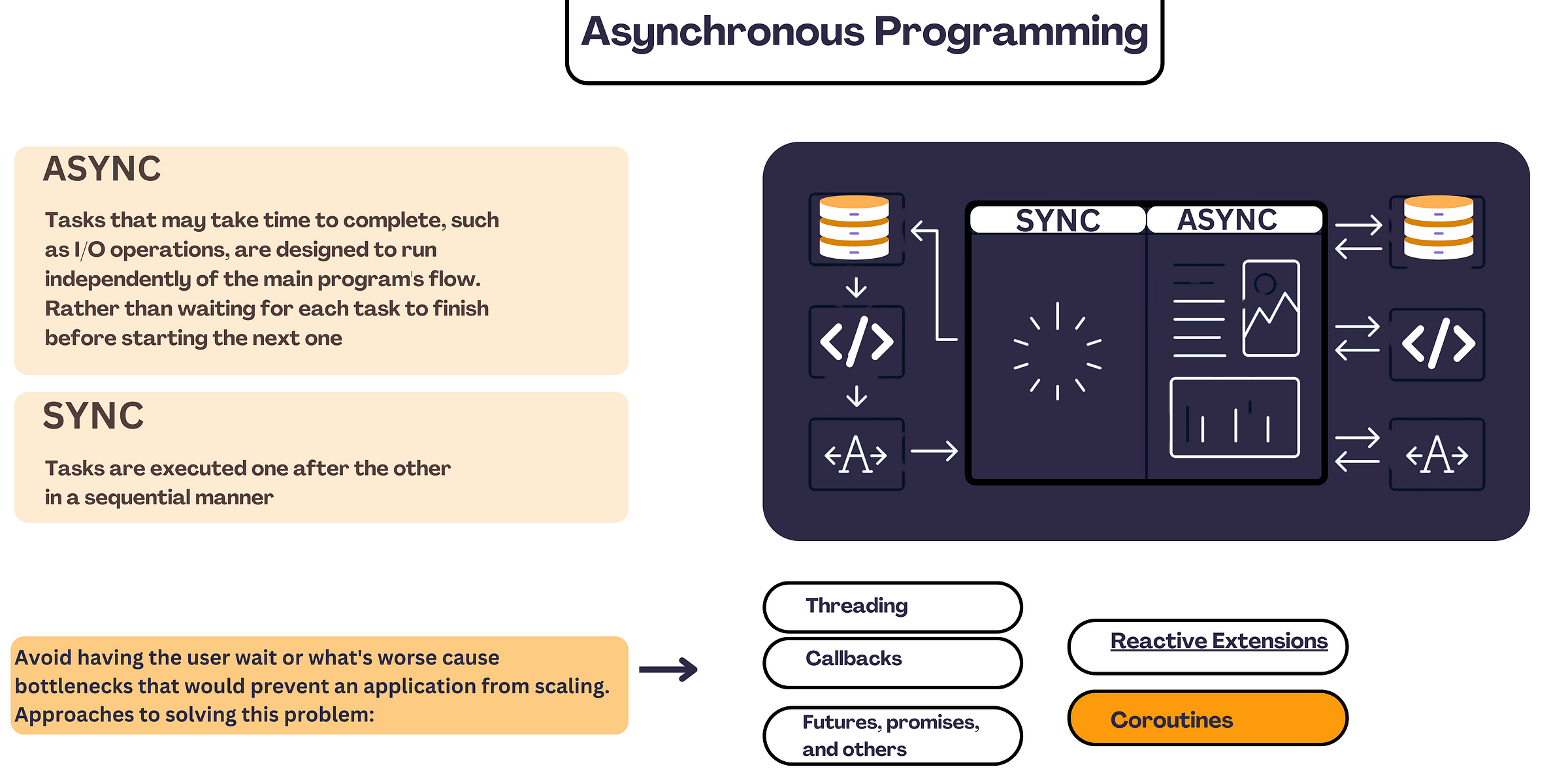
Table of Contents
What is asynchronous vs synchronous programming?
In computer programs, we often have tasks that take time, like fetching data from the internet or reading a file. In synchronous programming, the program would stop and wait for each of these tasks to complete before moving on. Asynchronous programming, however, lets the program start a task and then continue doing other things while waiting for the task to finish.
Imagine you’re playing a game on your computer, and you want to save your progress. With asynchronous programming, the game doesn’t freeze while it’s saving; you can still move your character or interact with the game world. The saving process happens in the background.
We aim to eliminate user wait times and prevent potential bottlenecks that hinder application scalability. Various solutions have been explored, such as:
- Threading
- Callbacks
- Futures, promises, and other techniques
- Reactive Extensions
- Coroutines
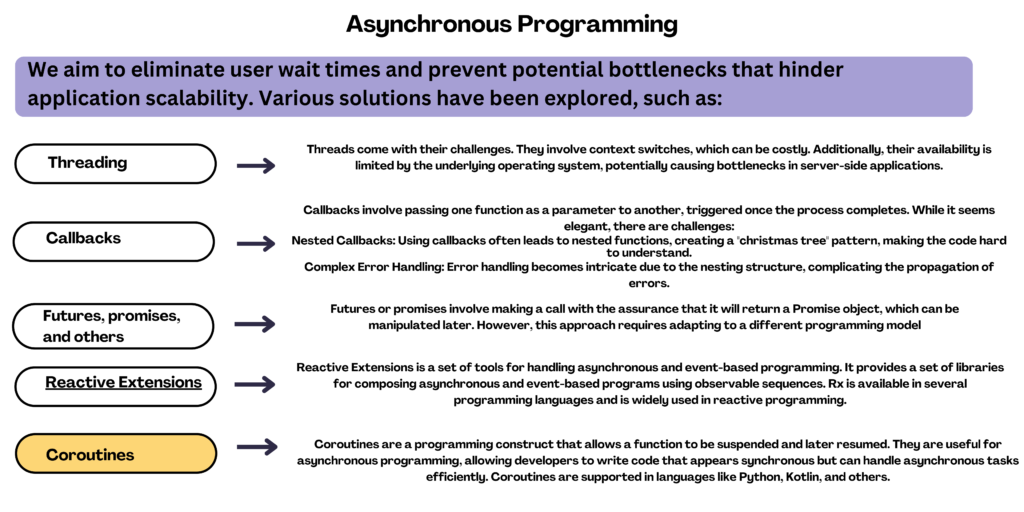
- Threading: Multitasking at Work Imagine you’re a manager handling various tasks simultaneously. Threading works similarly, allowing your app to handle multiple jobs without waiting for one to finish before starting another.
// Real-life code example
val thread = Thread {
println("Task 1: Processing...")
Thread.sleep(2000)
println("Task 1: Done!")
}
// Start the thread
thread.start()
KotlinExplanation:
val thread
: We’re creating a new thread, like assigning a new task to an assistant.Thread { ... }
: The code inside the curly braces represents the task the assistant will perform.println("Task 1: Processing...")
: Imagine your assistant announcing they’re starting a task.Thread.sleep(2000)
: Simulates the task taking 2 seconds.println("Task 1: Done!")
: After 2 seconds, the assistant announces the completion of the task.
- Callbacks: A Phone Call for Updates Think of callbacks as leaving a voicemail and getting a call back when the recipient is ready to talk.
// Real-life code example
fun fetchData(callback: (String) -> Unit) {
val data = "Fetched data"
callback(data)
}
// Usage
fetchData { result ->
println("Data received: $result")
}
KotlinExplanation:
fun fetchData(callback: (String) -> Unit)
: We’re creating a function that fetches data and calls back with the result.val data = "Fetched data"
: Simulates getting the data.callback(data)
: The callback function is like returning a call with the fetched data.fetchData { result -> println("Data received: $result") }
: We’re using the function and providing a callback that prints the received data.
- Futures and Promises: Ordering Food Online Picture placing an order online, getting an order number (promise), and later exchanging it for your meal (result).
// Real-life code example
val futureResult: Future<String> = // Simulate a future result
// When ready, get the result
val result = futureResult.get()
println("Result: $result")
KotlinExplanation:
val futureResult: Future<String>
: We’re creating a placeholder for a future result.val result = futureResult.get()
: When the result is ready, it’s like picking up your order.println("Result: $result")
: Printing the received result.
- Reactive Extensions: Subscribing to Events Imagine subscribing to a magazine and being notified whenever a new issue arrives.
// Real-life code example
Observable.create<String> { emitter ->
emitter.onNext("Event occurred")
}.subscribe { event ->
println("Received event: $event")
}
KotlinExplanation:
Observable.create<String> { emitter -> ... }
: Creating an observable, like subscribing to events.emitter.onNext("Event occurred")
: Emitting an event, like receiving a new magazine issue..subscribe { event -> println("Received event: $event") }
: Reacting to the event, or reading the new magazine issue.
What is an example of asynchronous programming technique?
In Kotlin, one common approach for asynchronous programming is using coroutines. Coroutines are lightweight threads that allow you to write asynchronous code in a more sequential and readable manner. Here’s a simple example demonstrating asynchronous programming with coroutines using the async
and await
functions:
import kotlinx.coroutines.*
suspend fun fetchData(): String {
delay(1000) // Simulating a network request or time-consuming operation
return "Data fetched successfully"
}
fun main() = runBlocking {
println("Start of the program")
// Using async to perform asynchronous operation
val deferredResult: Deferred<String> = async {
fetchData()
}
// Do other work while waiting for the asynchronous operation to complete
println("Performing other tasks...")
// Using await to get the result of the asynchronous operation
val result = deferredResult.await()
// Continue with the result
println("Result: $result")
println("End of the program")
}
KotlinIn this example, the fetchData
function simulates an asynchronous operation using the delay
function. The async
coroutine builder is used to perform the asynchronous operation, and await
is used to retrieve the result once it’s ready. Meanwhile, other tasks can be performed while waiting for the asynchronous operation to complete.
What is asynchronous programming in Android?
Asynchronous programming in Android involves executing tasks in the background to keep the UI responsive. Various tools and techniques, such as AsyncTask, Handlers, Threads, Java Executor Framework, and Kotlin Coroutines, can be employed based on the specific requirements and preferences of the developer.
Feel free to follow and join my email list at no cost. Don’t miss out — sign up now!
Please check out my earlier blog post for more insights. Happy reading!
The Innovative Fundamentals of Android App Development in 2023