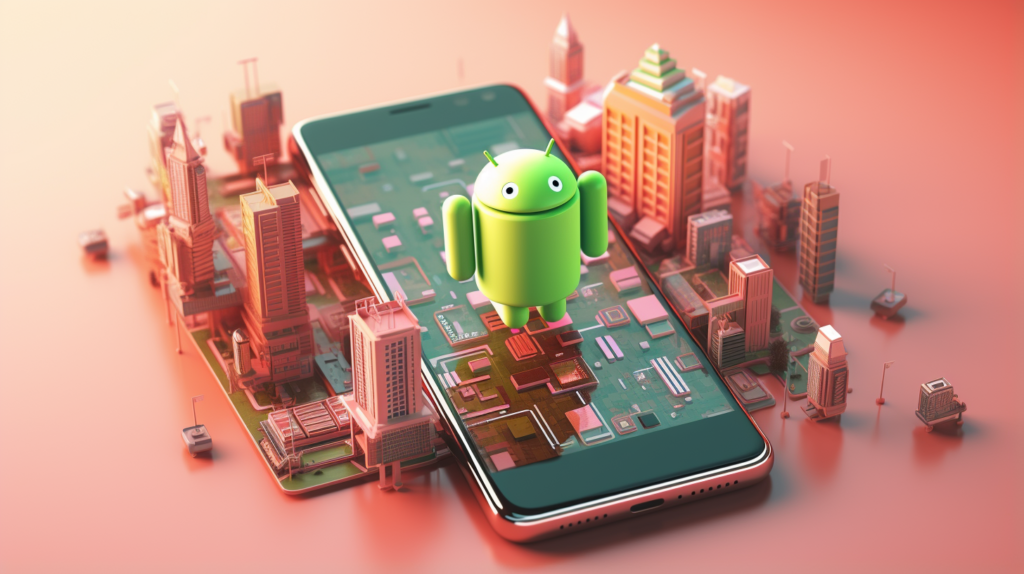
Table of Contents
Ever started building an Android app and stumbled upon the word “ViewModel”? Don’t worry if it sounds like tech-talk from another planet. Think of ViewModel as your app’s expert organizer for handling data, even when your screen flips or things change. We’re here to guide you, starting from scratch, and help you make sense of ViewModel’s magic. By the end, you’ll be confidently using it to build better apps. Ready to dive in? Let’s go!
I will assume that you know nothing about Android ViewModel, and then I will use more technical words to explain the ViewModel
What’s the Deal with Android ViewModel?
Alright, so here’s the scoop: Android ViewModel is like a special tool that helps each game remember stuff without getting mixed up. Just like how you use folders to keep your drawings organized, ViewModel keeps important information safe and neat for each game.
Let’s say you’re playing that awesome puzzle game. You’ve solved a bunch of levels, and suddenly your friend calls you to play a different game. No problem! Android ViewModel makes sure that the puzzle game remembers exactly where you left off and all the levels you’ve solved. It’s like magic!
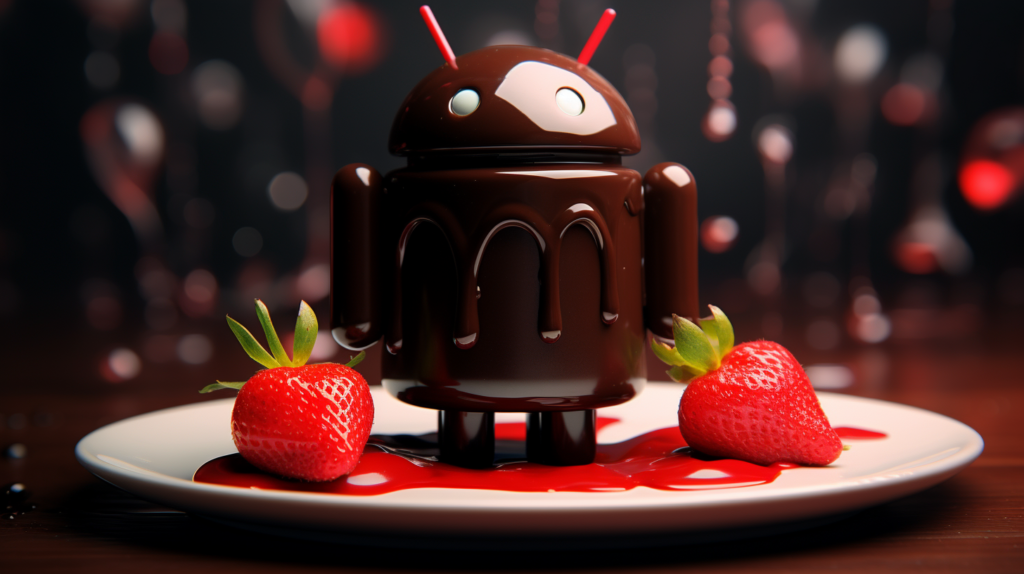
Why Do We Need ViewModel ?
You might wonder, “Why not just let the game remember stuff on its own?” Well, sometimes games take breaks or need to switch to a different part of the playground. When this happens, the ViewModel steps in and says, “Don’t worry, I’ll keep track of everything for you!”
So, when you come back to your puzzle game after hanging out with your friend, the ViewModel helps the game remember everything, just like when you press pause in a game and then continue later.
One ViewModel for Each Game
Here’s the cool part: every game has its own special ViewModel. This means that even if you’re playing two different games, like the puzzle game and the coloring app, they won’t mix up their memories. Each game has its own super memory helper!
What is the difference between view and ViewModel in Android?
Imagine you’re creating a painting – you have the canvas (which is like the screen of your phone) where you’ll put all the colors and shapes to make your picture (which is your app). In this scenario, the “view” is like the canvas; it’s what people see on the screen, like buttons, images, and text. It’s where everything visually happens.
Now, think of the “ViewModel” as your personal helper who makes sure your colors and shapes stay organized while you’re painting. This helper knows how to keep things neat, even if you need to take a break or if someone turns your canvas around. They remember where each color goes and how to fix things if something changes.
So, in simple words, the “view” is what you see on the screen, and the “ViewModel” is the behind-the-scenes friend that helps keep everything in order and makes sure your app looks and works just the way you want it to, no matter what happens.
Aspect | View | ViewModel |
---|---|---|
Purpose | Handles UI presentation and interaction | Manages UI-related data and state |
Responsibility | Displays UI elements (e.g., buttons, text) and handles user input events | Holds data and business logic for the UI, enabling separation of concerns |
Lifecycle Awareness | Aware of Android’s lifecycle (Activity or Fragment) | Also lifecycle-aware, but exists independently of UI components |
Data Binding | Can be connected with ViewModel for dynamic updates | Can be observed by View for dynamic updates using LiveData |
Data Management | Doesn’t manage data, just presents it | Manages data, maintains state, and prepares data for the View |
State Preservation | Doesn’t inherently retain data across configuration changes | Retains data across configuration changes, preventing data loss |
Communication | May require callbacks or interfaces for data communication | Facilitates communication between UI components using shared ViewModels |
Testing | Can be harder to test due to direct interaction with UI elements | Easier to test, as business logic can be tested independently |
Memory Management | Holds references to UI components, potentially leading to memory leaks | Doesn’t hold references to Views, reducing memory leak risks |
Example | Button, TextView, ImageView, etc. | ViewModel for a weather app managing temperature data and forecast logic |
What is a ViewModel in Android?
ViewModel is a class is a Android Architecture Components and you may asking now what is that? it is designed to store and manage UI-related data .You can think that Architecture Components is bridge between the UI (Activity or Fragment) and the underlying data sources, such as databases, repositories, or network requests.
What is the benefit of ViewModel in Android?
ViewModels in Android provide lifecycle-aware data separation, persistence, and testing benefits, enhancing UI management and performance.

How to create a ViewModel in Android?
Creating a ViewModel in Android using Hilt, Compose, and Kotlin involves several steps. I’ll be honest with you; the setup part looks confusing at first, but once you set it up a couple of times, you’ll start memorizing the process. Please be careful with the dependency part, as you might need to use a newer version. I’ll leave the link to the official documentation. Let’s get started!
Setup Dependencies:
Make sure you have the necessary dependencies in your app-level build.gradle
file:
implementation "androidx.lifecycle:lifecycle-viewmodel-compose:1.0.0-alpha07"
implementation "androidx.lifecycle:lifecycle-runtime-ktx:2.4.0-beta01"
implementation "com.google.dagger:hilt-android:2.40.5"
kapt "com.google.dagger:hilt-android-compiler:2.40.5"
implementation "androidx.hilt:hilt-navigation-compose:1.0.0-alpha03"
KotlinCreate a ViewModel:
Create a Kotlin class for your ViewModel. It should extend ViewModel
from the androidx.lifecycle
package. Define your data and methods that manage that data here.
import androidx.lifecycle.ViewModel
import dagger.hilt.android.lifecycle.HiltViewModel
import javax.inject.Inject
@HiltViewModel
class MyViewModel @Inject constructor() : ViewModel() {
// Define your ViewModel's data and methods here
}
KotlinSetup Hilt for ViewModel Injection:
Annotate your ViewModel with @HiltViewModel
to enable Hilt to inject dependencies into it.
Inject ViewModel into Composable:
In your Composable function, you can use the hiltViewModel()
function from androidx.hilt.navigation.compose
package to obtain an instance of your ViewModel. This function will automatically provide the correct ViewModel instance tied to the current Composable’s lifecycle.
@Composable
fun MainComposeLayout() {
val viewModel: MainViewModel = hiltViewModel()
Button(onclick = { viewModel.onButtonClicked() }) {
Text("Call To Action")
}
}
Kotlin
Should every view have a ViewModel?
Not necessarily. The decision to use a ViewModel for a particular View depends on various factors and the complexity of your app.
ViewModels are particularly beneficial when:
- Data Persistence Across Configuration Changes: If a View needs to retain its data and state across configuration changes (like screen rotations), using a ViewModel can ensure that the data remains intact.
- Business Logic and Data Management: If a View involves complex data operations or business logic, a ViewModel can help separate this logic from the View itself, leading to cleaner and more maintainable code.
- Shared Data: When multiple fragments within an activity need to share data or communicate with each other, using a ViewModel can provide a centralized way to manage and share that data.
- Testing: If you want to unit test your View’s logic without dealing with Android’s UI components, using a ViewModel can make testing easier and more effective.
However, for simple Views that don’t involve much data or logic, introducing a ViewModel might not provide significant benefits and could add unnecessary complexity. The decision to use a ViewModel should be based on your app’s architecture, the specific needs of your Views, and your overall design goals. It’s important to find the right balance between using ViewModels to enhance separation of concerns and avoiding over-engineering.

Is ViewModel part of UI?
No, ViewModel is not a direct part of the UI. ViewModel is a component of the Android Architecture Components that is designed to store and manage UI-related data. It acts as a bridge between the UI components (such as activities and fragments) and the underlying data sources like databases or repositories.
ViewModel’s primary purpose is to separate the UI logic from the data and business logic. It helps in managing the UI-related data and state in a way that survives configuration changes (like screen rotations) without causing memory leaks or data loss. While ViewModel plays a crucial role in enhancing the UI experience, it operates behind the scenes and focuses on data management rather than directly controlling the user interface.
Relationship between ViewModel and the Android UI components (Activity/Fragment)
Think of your app like a play. You have actors on the stage, and the audience watches them. The actors represent the Android UI components – Activities and Fragments. They’re the ones you see on the screen.
Now, behind the scenes, you have a script and cues for the actors to follow. This script is like the data and logic your app needs to work. Here’s where the ViewModel steps in. Imagine the ViewModel as the director giving cues to the actors.
The actors (UI components) don’t directly handle the script (data and logic). They talk to the director (ViewModel), and the director knows what data each actor needs and when to give it to them. It’s like the ViewModel holds the backstage instructions.
Now, sometimes the play changes. Maybe the stage rotates or the lights go off. But the director (ViewModel) remembers everything and ensures that when the play continues, the actors have the right cues and instructions, no matter what happened.
In short, the ViewModel is like the director that manages the data and instructions for the actors (UI components) in your app. It keeps things organized, prevents confusion, and ensures a smooth performance for your audience (users).
When should I use ViewModel Android?
Remember that while ViewModel
can be very helpful in many scenarios, it might not be necessary for every use case. Simple UIs or cases where you don’t need to manage UI-related data across lifecycle events might not require a ViewModel
. It’s important to understand your app’s requirements and architecture before deciding when and where to use the ViewModel
class.
What is the difference between ViewModel and LiveData in Android?
In Android development, both the ViewModel
and LiveData
components play essential roles in creating robust and maintainable applications. The ViewModel
serves as a container for UI-related data, ensuring its persistence through configuration changes and the recreation of UI components. This separation of concerns promotes cleaner code architecture. On the other hand, LiveData
acts as an observable data holder, seamlessly integrating with UI elements. Its lifecycle-aware nature guarantees that UI components only display the most recent data when active and automatically halts updates when inactive, addressing potential memory leak concerns. These two components work harmoniously: the ViewModel
manages data, and LiveData
facilitates efficient data flow to the UI, collectively enhancing the structure and responsiveness of Android applications.
Feature | ViewModel | LiveData |
---|---|---|
Purpose | Manages and retains UI-related data | Provides lifecycle-aware data updates |
Data Management | Holds UI-related data across lifecycles | Observes changes in data and updates UI accordingly |
Lifecycle Awareness | Not lifecycle-aware by default, but can be observed | Lifecycle-aware, updates UI only when active |
Data Sharing | Supports sharing data between UI components | Facilitates data propagation to UI layer |
Separation of Concerns | Isolates UI logic from UI components | Ensures UI updates based on data changes |
Memory Management | Can help prevent memory leaks by allowing controlled release of resources | Automatically removes observers to prevent leaks |
Dependency | Not directly dependent on UI lifecycle | Tightly integrated with UI lifecycle |
Unit Testing | Easily testable due to separation from UI | Requires testing with respect to lifecycle events |
Use Case | Recommended for managing UI data and state | Recommended for exposing data changes to UI |
Interview questions related to ViewModel in Android
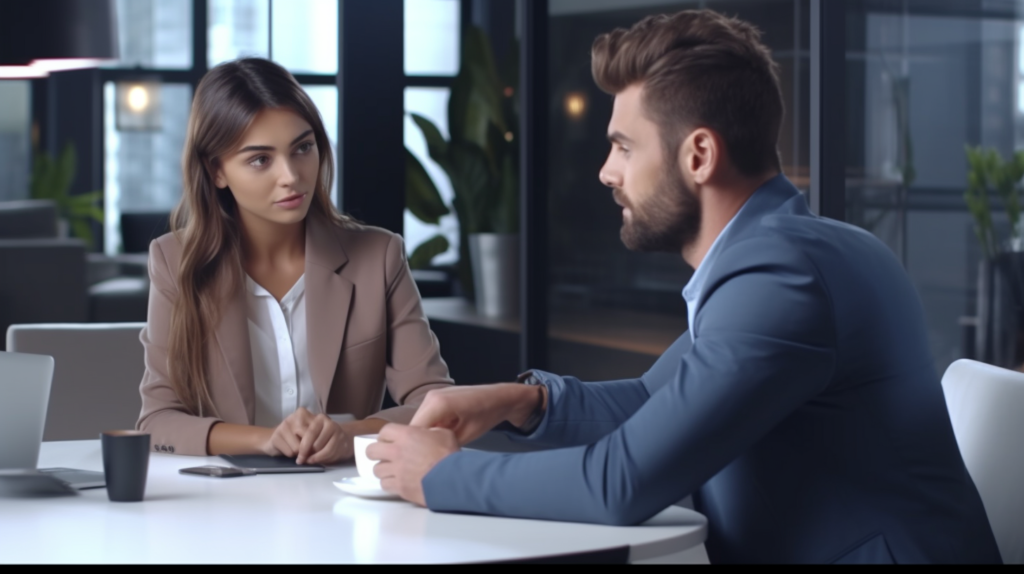
Interview questions related to ViewModel in Android
During an Android development interview, you might encounter questions related to Android ViewModel. You could be asked to explain the role of ViewModel in app development and its ability to tackle data persistence across configuration changes. You might need to elaborate on how ViewModel collaborates with UI components like Activities and Fragments and elucidate the relationship between ViewModel and LiveData. Interviewers might inquire about the importance of refraining from holding Views or Contexts in ViewModels, and the ways to share data between fragments using ViewModel.
- What is Android ViewModel, and what problem does it solve in app development?
- Explain the relationship between ViewModel and the Android UI components (Activity/Fragment).
- How does ViewModel preserve data during configuration changes, such as screen rotations?
- What’s the difference between ViewModel and LiveData? How do they work together?
- Why is it recommended not to hold references to Views or Contexts inside a ViewModel?
- How can you share data between fragments using ViewModel?
What is the Best Way to Learn Web Development in 2023?
The Innovative Fundamentals of Android App Development in 2023
How to learn Android App Development in 2023 – Full Guide For Beginners using Kotlin Language
You can get full access to every story on Medium for just $5/month by signing up through this link