
Table of Contents
What is the Repository Pattern in Android?
The Repository Pattern in Android is a design approach that provides a structured and organized way to manage data access and manipulation. It serves as an intermediary between different data sources (like databases, network services) and the rest of the application. The primary goal is to abstract the data access details, promoting clean architecture, code reusability, and maintainability.
Repository Pattern Key Components:
- Data Models: Define the structure of the data entities you’ll be working with (e.g., User, Task).
- Data Sources: Implement classes that interact with specific data sources (e.g., Room database, Retrofit for network requests).
- Repository: Create a repository class that acts as a bridge between data sources and the rest of the app. It provides methods for data access and manipulation.
Repository Pattern Example Use Case:
For instance, in a “Notes App,” a repository can manage interactions with notes. It fetches notes from the database if available, otherwise from a network source. The ViewModel interacts with the repository to provide notes to the UI.
In essence, the Repository Pattern offers a consistent, modular approach to handling data in Android apps. It enhances code organization, reusability, and maintainability while promoting a clear separation of concerns.
What do you mean by design pattern?
Imagine you’re building a house. When architects design houses, they follow certain guidelines and strategies that make the houses comfortable, efficient, and functional. These guidelines are like design patterns for houses.
In the same way, when software developers create computer programs, they face common problems like how to organize code, how different parts of the program should work together, and how to make the program easy to change in the future. Design patterns are like these helpful guidelines for software. They are tried-and-tested solutions that experienced programmers have come up with to solve these common problems.
Just like architects have blueprints to build houses, programmers have design patterns to build software. These patterns are not ready-made pieces of code, but rather ways of thinking and organizing code so that the software is reliable, easy to understand, and can be modified if needed. They help programmers communicate better, work more efficiently, and create high-quality software just like architects create well-designed houses.
What are the three main design patterns?
Category | Design Patterns |
---|---|
Creational | Singleton, Factory Method, Abstract Factory, Builder |
Structural | Adapter, Composite, Decorator, Facade, Proxy, Bridge |
Behavioral | Observer, Strategy, Command, Template Method, State, Interpreter, Chain of Responsibility, Mediator, Visitor, Memento |
Please note that this table includes only a subset of the design patterns, and there are additional design patterns beyond those listed here. Each of these patterns serves a specific purpose in software design and architecture.
What is the purpose of repository?
The purpose of a repository, in the context of software development and databases, is to provide a standardized way to manage and interact with data stored in a persistent storage system, such as a database. The Repository Pattern is a design pattern that helps separate the data access code from the rest of the application’s business logic. It serves several important purposes

What is a Repository Pattern?
The Repository Pattern is a design pattern used in software development to abstract and isolate the data access layer of an application from the rest of the application’s code. It provides a structured and consistent way to interact with data storage systems such as databases, APIs, and external services. The main purpose of the Repository Pattern is to encapsulate the details of data storage and retrieval, making the codebase more maintainable, modular, and testable.
How to create Repository in Android?
Here’s a general outline of how you can create a repository in Android using Kotlin:
- Define Data Models: Define the data models that your app will use. These models represent the entities you’ll be working with, such as users, items, or any other data your app needs to manage.
- Create Data Sources: Implement the data sources that the repository will interact with. This can include local data sources (e.g., Room database) and remote data sources (e.g., Retrofit for network requests).
- Create the Repository: Create a repository class that acts as an interface between the ViewModel and the data sources. This class should provide methods to access and manipulate data. You can include methods for fetching, inserting, updating, and deleting data.
- Implement Data Access Logic: Inside the repository, implement the data access logic using the data sources you defined earlier. Depending on your use case, you might need to handle data transformation, caching, and error handling.
- Expose Repository Methods: Expose the repository methods you’ve implemented to be used by the ViewModel. You can expose these methods as
suspend
functions if they involve asynchronous operations.
class UserRepository(private val userDao: UserDao, private val apiService: ApiService) {
// Fetch users from the data sources (local database and network)
suspend fun getUsers(): List<User> {
val cachedUsers = userDao.getAllUsers()
return if (cachedUsers.isNotEmpty()) {
cachedUsers
} else {
val networkUsers = apiService.fetchUsers()
userDao.insertUsers(networkUsers)
networkUsers
}
}
// Other repository methods for data manipulation (insert, update, delete) can be added here
}
KotlinIn this example, UserRepository
uses a local database (userDao
) and a network service (apiService
) to fetch users. If local data is available, it returns that data; otherwise, it fetches data from the network and stores it locally.
What is a Repository in MVVM?
Android development using Kotlin and following the MVVM (Model-View-ViewModel) architectural pattern, a repository is a component that serves as an intermediary between the ViewModel and the data sources, such as a local database, network services, or other data storage mechanisms. The repository’s main purpose is to manage data access operations and abstract away the details of how data is fetched and manipulated.
Here’s a simplified breakdown of how a repository fits into MVVM architecture in Android using Kotlin:
- Model Layer: The Model layer consists of the data models and the repository. The repository acts as a bridge between the ViewModel and the data sources.
- ViewModel Layer: The ViewModel is responsible for handling UI-related logic, managing the UI state, and exposing data to the UI components. It interacts with the repository to fetch and manipulate data.
- View Layer: The View layer consists of UI components, such as Activities, Fragments, and Layouts. It observes the ViewModel’s data changes and updates the UI accordingly.
Why use the Repository Pattern?
Not using the repository pattern can result in scattered, duplicated, and tightly coupled data access code. This can lead to inconsistent behavior, testing challenges, and increased maintenance complexity. Changes to data sources or logic become harder and debugging becomes complex. The lack of a standardized approach hampers flexibility, hindering adaptation and cross-platform support. Collaboration difficulties arise, slowing development and increasing the learning curve for new developers. In contrast, the repository pattern organizes data access, reduces code duplication, and separates concerns for cleaner, maintainable, and adaptable code, fostering collaboration and efficient development.
What is the difference between DAO and Repository?
DAO (Data Access Object):
- DAO deals with direct database interactions and specific SQL queries.
- It’s like a helper that talks directly to the database to get or change data.
- Focuses on low-level operations, like reading and writing data in the database.
Repository:
- Repository manages how data is accessed, whether from databases, network, or other sources.
- It’s like a manager that handles getting, storing, and manipulating data for the rest of the app.
- Focuses on simplifying data access for the app and can work with various data sources.
In simple terms, DAO is about talking to the database, while a repository is about managing data for the whole app from various sources.
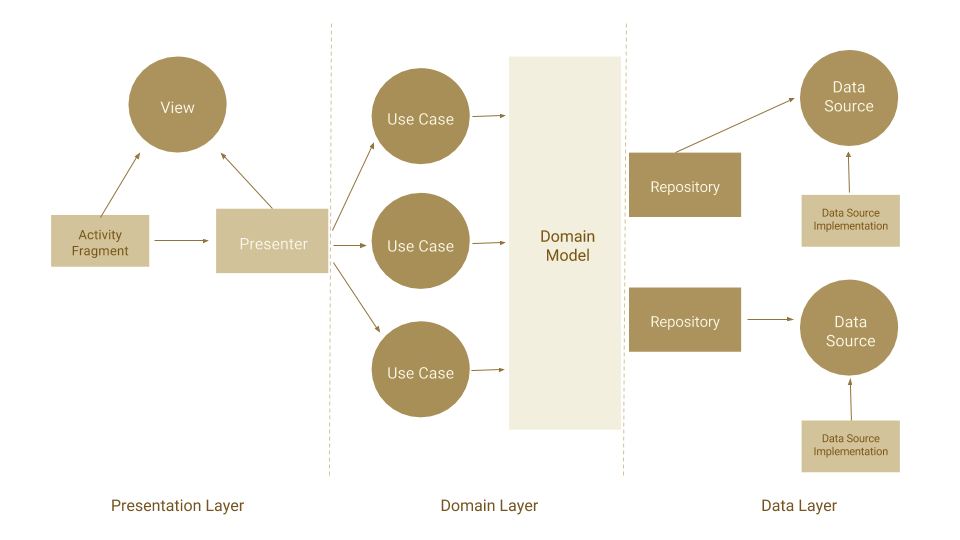
Please check out my earlier blog post for more insights. Happy reading!
The Innovative Fundamentals of Android App Development in 2023
How to learn Android App Development in 2023 – Full Guide For Beginners using Kotlin Language
How to be Productive When Working From Home
Ready to level up your software skills? Follow and Join my email list for free insights on software and Android development, plus valuable tips for personal growth. Don’t miss out — sign up now!
I could not refrain from commenting. Exceptionally well written!