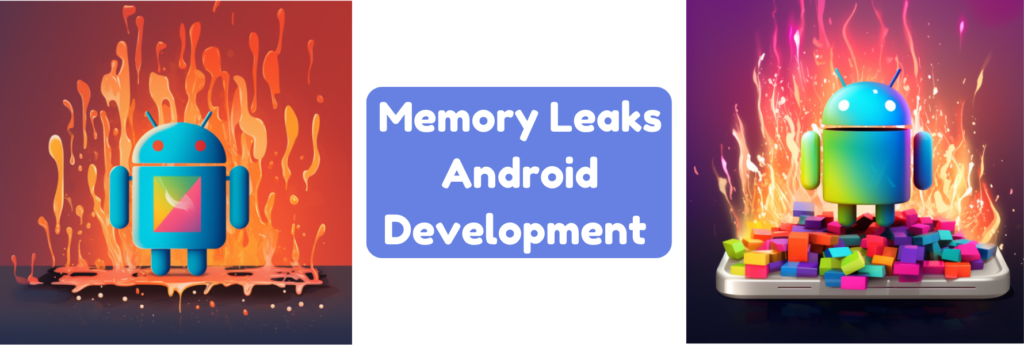
I’m putting together this blog post because I realized that my previous one about “The 5 Most Common Android Memory Leaks” might not have been the most beginner-friendly. I want to take a step back and explain memory leaks for everyone, especially those who are new to Android development. Trust me, grasping this topic is super important, and I want to make sure it’s crystal clear for all of you. Let’s dive into the world of memory leaks together!
Table of Contents
What is memory leaks and how do you solve it in Android?
Imagine your brain is like a bookshelf, and you have books on it. These books represent the things your computer is working on. Now, when you’re done with a book, you’re supposed to put it back on the shelf so you have room for new books. But sometimes, you might forget to put a book back, and it stays on your desk.
Similarly, in a computer, there’s a part called “memory” that’s like the desk space for the computer’s work. When a computer program finishes using something, it’s like finishing a task and being done with a book. The program should “put away” or release that memory so it can do new tasks with fresh memory space.
However, just like if you don’t put your books back on the shelf, the desk can get messy, computer programs can sometimes forget to release memory they no longer need.
This is called a memory leak. Over time, these unused bits of memory can pile up and slow down the program or even make it crash.
Solving a memory leak is like cleaning up your desk.
You need to identify those forgotten books (unused memory), put them back on the shelf (release the memory), and make room for new tasks. Computer experts use tools to find these memory leaks and fix them, just like you might tidy up your desk to have a neat and organized workspace.
Essential technical terms related to Android memory leaks
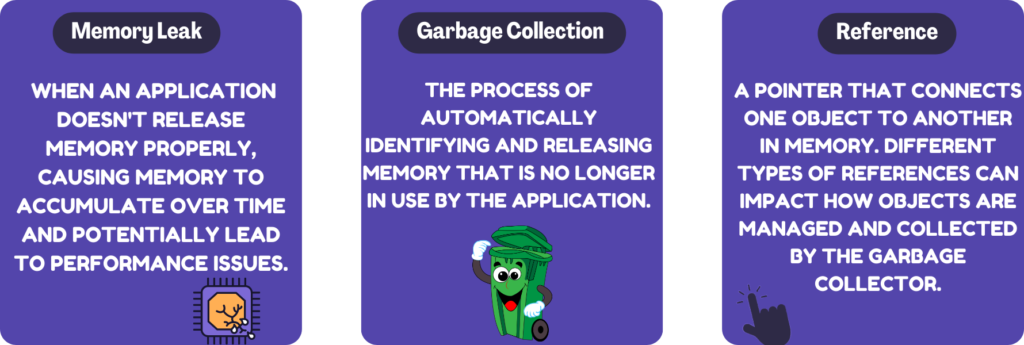
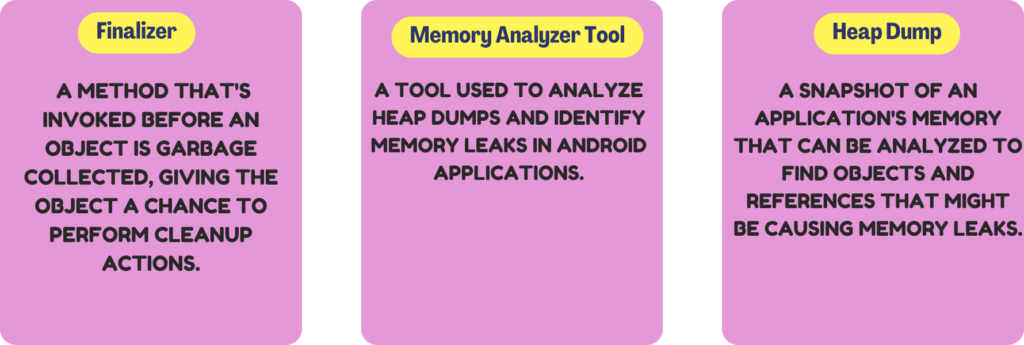
How can I fix a Memory leaks?
Fixing a memory leak involves identifying the root cause of the leak and implementing appropriate solutions. Here’s a step-by-step guide to help you fix a memory leak in your Android application:
- Identify the Leak: Use tools like the Android Profiler or LeakCanary to identify which parts of your code are causing the memory leak. These tools can provide you with valuable information about the objects and references that are not being properly released.
- Analyze the Code: Once you identify the leak, review the relevant code to understand why the memory is not being properly released. Look for places where objects are being retained longer than necessary or where references are not being properly cleared.
- Release References: Identify the objects and references that are causing the leak and make sure to release them when they are no longer needed. For instance:
- Unregister listeners and callbacks.
- Clear references to activities and fragments when they are destroyed.
- Dispose of resources like streams, databases, and network connections when they’re no longer required.
- Use Weak References: In cases where you need to hold references, consider using weak references. Weak references allow the garbage collector to collect the object if there are no strong references to it. However, be cautious with weak references, as they have limitations and might not always fit your use case.
- Check Threading and Handlers: Ensure that you’re properly managing threads and handlers. Make sure to stop or terminate threads and handlers when they are no longer needed. Threads and handlers can easily cause memory leaks if they’re not managed correctly.
- Optimize Bitmap Handling: If your application deals with images, optimize the handling of bitmaps. Use appropriate scaling techniques and consider caching strategies to prevent excessive memory consumption.
- Review Custom Views and View Hierarchies: If you’re using custom views, double-check that they aren’t inadvertently holding references to their parent contexts or other objects. Also, be mindful of view hierarchies and make sure to clean up any unnecessary views.
- Test Thoroughly: After implementing fixes, thoroughly test your application to ensure that the memory leak has been resolved. Use tools like LeakCanary and the Android Profiler to verify that the memory leak no longer occurs.
- Monitor and Maintain: Continuously monitor your application for memory leaks, especially as you make changes or introduce new features. Regularly review and analyze memory usage to catch any potential leaks early.
- Documentation and Code Reviews: Document the fixes you implemented to address the memory leak. Additionally, conduct code reviews with your team to ensure that memory leak prevention strategies are followed consistently.
Remember that fixing memory leaks can sometimes involve trial and error. It’s important to test thoroughly and be vigilant in your approach to ensure that the leak is fully resolved without introducing new issues.

What causes a Memory leak?
Memory leaks can occur due to various coding practices and scenarios in your daily development work. Here are some common causes of memory leaks that you might encounter while coding:
- Unclosed Resources: Failing to close resources like files, streams, database connections, or network connections after using them can lead to memory leaks. These resources consume memory that’s not being released.
- Listeners and Callbacks: Registering listeners or callbacks without properly unregistering them can cause objects to be retained in memory even when they’re no longer needed.
- Retained References: Holding onto references to objects longer than necessary can prevent the garbage collector from reclaiming memory. This often happens with static references or references held by long-lived objects.
- Activity and Fragment Lifecycle: Not properly releasing resources or references when an activity or fragment is destroyed can result in memory leaks. If references to these objects are held elsewhere, they’ll prevent the garbage collector from collecting them.
- Thread Mismanagement: Incorrectly managing threads and not stopping them when they’re no longer needed can lead to memory leaks. Threads that are still running can hold onto objects and prevent their cleanup.
- Custom Views and View Hierarchies: Custom view components can inadvertently hold references to their parent context or other objects, preventing the proper garbage collection of those objects.
- Caching: Improper caching mechanisms can lead to memory leaks. Cached objects might not be properly released, causing unnecessary memory consumption.
- Strong References in Closures: Be cautious when using closures or anonymous inner classes. If they hold references to surrounding objects, those objects might not be garbage collected.
- Static Variables: Holding references to objects in static variables can lead to memory leaks, especially if those objects are no longer needed but the static variable still holds a reference to them.
- Long-lived Objects: Long-lived objects like singletons or instances held in global contexts can accumulate references and lead to memory leaks if not managed properly.
- Implicit References: Be aware of any implicit references that certain libraries or frameworks might introduce. These references can lead to objects being retained longer than expected.
- Failure to Implement
onDestroy
oronStop
: In Android, not properly implementing lifecycle methods likeonDestroy
oronStop
can prevent resources from being released and cause memory leaks.
Preventing memory leaks involves careful coding practices, regular testing, and a good understanding of memory management principles. Being mindful of the objects you’re creating, retaining, and releasing will help you avoid memory leak pitfalls in your daily coding tasks.
What is the main cause of memory leaks in Android?
Let’s chat about a common challenge we face in our coding adventures: memory leaks. Picture this: you’re coding away, creating awesome features for your app, and suddenly, you notice something isn’t quite right. Your app’s performance is sluggish, and crashes seem to be making a guest appearance more often than you’d like. Well, my friend, you might just be dealing with a memory leak. So, what’s the scoop? Memory leaks in Android typically happen when we forget to tidy up after ourselves. You know, not closing those file streams, not saying goodbye to callbacks, or holding onto references longer than a weekend hangout. We’re talking about unclosed resources, forgotten listeners, and even threads that just won’t quit. It’s like leaving your room a mess after a gaming session – stuff just piles up, and the space becomes unusable! But fret not, because there’s a silver lining. By being vigilant with resource cleanup, unregistering those listeners, and knowing when to let go of references, we can put an end to these sneaky leaks. So let’s roll up our sleeves, dive into our code, and make sure our apps are leak-free zones where memory can roam freely without worry!
What is the best tool to detect memory leaks?
When it comes to detecting memory leaks in Android applications, one of the most widely recommended and used tools is LeakCanary. LeakCanary is an open-source library developed by Square, designed specifically to help you identify and track down memory leaks in your Android app.
LeakCanary is known for its simplicity and effectiveness in detecting memory leaks. It operates as a lightweight library that you can integrate into your project with minimal effort. Here’s why LeakCanary is often considered the best tool for detecting memory leaks:
- Easy Integration: Adding LeakCanary to your project is straightforward. You simply add the library to your app’s dependencies, and it takes care of the rest.
- Automatic Leak Detection: LeakCanary automatically monitors your app for memory leaks in the background, so you don’t have to manually trigger tests. It notifies you when a memory leak is detected, making it easy to catch issues early.
- Detailed Reports: When a memory leak is detected, LeakCanary provides detailed reports that pinpoint the exact location and cause of the leak. This information includes the leaking object, the path to the GC root, and the context in which the leak occurred.
- Visualized Data: LeakCanary’s reports are visually informative, helping you understand the structure of the leaked object’s references and where it’s being retained.
- Integration with Android Studio: LeakCanary integrates seamlessly with Android Studio, making it even more convenient to detect and analyze memory leaks during development.
- Customization: LeakCanary provides options to customize its behavior, like setting up different thresholds for leak detection, which can be useful in tailoring the tool to your app’s specific needs.
- Community Support: Since LeakCanary is widely used in the Android development community, you can find ample resources, tutorials, and discussions to help you make the most of the tool.
While LeakCanary is a fantastic tool, it’s important to note that no tool is a one-size-fits-all solution. Depending on your specific app and requirements, you might also consider using other memory profiling tools like the Android Profiler, MAT (Memory Analyzer Tool), or your own custom logging and monitoring solutions. However, LeakCanary remains an excellent starting point for detecting memory leaks in your Android applications.
Feel free to follow and join my email list at no cost. Don’t miss out — sign up now!
Please check out my earlier blog post for more insights. Happy reading!
The Innovative Fundamentals of Android App Development in 2023
How to learn Android App Development in 2023 – Full Guide For Beginners using Kotlin Language
Ultimate Guideline on How to Use ViewModel Design Pattern
What is the Repository Pattern in Android? A Comprehensive Guide
What is ViewModel in Android? A Good Guide for Beginners
The Innovative Fundamentals of Android App Development in 2023
How to Say Goodbye to Activity Lifecycle and Say Hello to Compose Lifecycle ?
How to Monitor/Observe Network Availability for Android Projects