
Table of Contents
What is infix functions in Kotlin?
Infix functions allow you to call functions with a single parameter in a clean and expressive manner, improving the readability of your code.
What are Infix Functions?
Infix functions are a feature in Kotlin that enable you to call functions with a single parameter using infix notation. This means you can call the function without using the traditional dot-notation or parentheses, making the code look more like a natural language expression.
Benefits of Infix Functions
- Readability: Infix functions enhance code readability by enabling you to create function calls that resemble plain English, making the code more intuitive and understandable.
- Conciseness: Infix functions allow you to write concise code, reducing the verbosity often associated with function calls.
- DSL (Domain-Specific Language) Creation: Infix functions are commonly used when creating internal DSLs in Kotlin, making the DSL code read like a series of natural language statements.
Implementing Infix Functions in Kotlin
1. Declaring an Infix Function
To declare an infix function in Kotlin, use the infix
keyword:
infix fun Int.add(x: Int): Int {
return this + x
}
Kotlin In this example, we’ve created an infix function named add
that takes an integer parameter and returns the sum of the caller and the parameter.
2. Using Infix Functions
Once you’ve declared an infix function, you can use it without the dot-notation or parentheses:
fun main() {
val result = 2 add 3 // Using infix function
println("Result: $result") // Output: Result: 5
}
KotlinHere, 2 add 3
is an infix function call, adding 2 and 3 together.
Let’s consider a concise and easy-to-read example using infix functions in the context of an Android project. This time, we’ll create a simple DSL for defining user profiles:
// DSL for defining user profiles
class UserProfileDSL {
data class UserProfile(var name: String = "", var age: Int = 0, var city: String = "")
// Infix function for setting the user's name
infix fun UserProfile.name(name: String) {
this.name = name
}
// Infix function for setting the user's age
infix fun UserProfile.age(age: Int) {
this.age = age
}
// Infix function for setting the user's city
infix fun UserProfile.city(city: String) {
this.city = city
}
}
// Example usage in an Activity or Fragment
fun main() {
// Using the DSL to define a user profile
val userProfile = UserProfileDSL().apply {
name "John Doe"
age 25
city "Example City"
}
// Printing the user profile
println("User Profile: $userProfile")
}
KotlinLet’s break down the code and explain it in detail for beginner developers.
- User Profile DSL Class (
UserProfileDSL
):UserProfileDSL
is a class representing a DSL (Domain-Specific Language) for defining user profiles. A DSL is a small, specialized language for a specific problem domain, in this case, creating user profiles.- Inside
UserProfileDSL
, there is a data class calledUserProfile
that represents the structure of a user profile with three properties:name
,age
, andcity
. - Three infix functions (
name
,age
, andcity
) are defined insideUserProfileDSL
. These functions are used to set the corresponding properties of aUserProfile
instance.
- Infix Functions (
name
,age
,city
):- Infix functions are special functions marked with the
infix
keyword. They allow for a more natural and readable syntax when calling them. In this case, they are used to set the properties of aUserProfile
. - The
name
function takes aString
parameter and sets thename
property of aUserProfile
instance. - The
age
function takes anInt
parameter and sets theage
property of aUserProfile
instance. - The
city
function takes aString
parameter and sets thecity
property of aUserProfile
instance.
- Infix functions are special functions marked with the
- Example Usage (
main
function):- The
main
function is like the entry point of the program, and it simulates an Android component (e.g., an Activity or Fragment). - An instance of
UserProfileDSL
is created:UserProfileDSL()
. - The DSL is used to define a user profile using the
apply
function, which allows us to configure the properties of theUserProfile
instance inside a block. - In the block, three infix function calls set the name, age, and city of the user profile.
- The resulting
UserProfile
instance is printed to the console, showing the values that were set.
- The
Interview Questions
What is an infix function in Kotlin?
Answer: An infix function in Kotlin is a function that is defined with theinfix
keyword and takes one parameter. It allows you to call the function using a special infix notation, making your code more readable and natural.
How is an infix function defined in Kotlin?
Answer: An infix function is defined by using theinfix
keyword before thefun
keyword in the function declaration. It should have one parameter.
What is the purpose of infix functions?
Answer: Infix functions are used to make code more readable and expressive by allowing you to call functions using a special infix notation. They are often used to create more natural and human-readable code, especially for operations that are commonly expressed as binary operations (e.g., mathematical operations, combining words).
Can infix functions only be used with mathematical operations?
Answer: No, infix functions can be used for various purposes beyond mathematical operations. While they are often used for operations like addition or subtraction, they can be applied to any situation where it makes code more readable and natural. For example, you can use them to concatenate strings, compare values, or perform custom operations.
Can infix functions only be defined in classes?
Answer: Infix functions can be defined in classes or as top-level functions. They are not limited to classes and can be defined in any Kotlin file.
Give an example of creating and using an infix function in Kotlin.
Answer: Provide a code example demonstrating the creation and usage of an infix function, such as adding two numbers or any other operation that illustrates the concept.
What are the restrictions on parameter types for infix functions?
Answer: Infix functions must have a single parameter, and the parameter type should be the same as the receiver type (the type on which the function is called). For example, if the infix function is called on anInt
, the parameter should also be anInt
.
Explain when you might use an infix function in a real-world programming scenario.
Answer: In a practical context, you can discuss scenarios where infix functions can improve code readability and make code more natural, such as creating custom operators for domain-specific operations or simplifying code for combining words or phrases.
Are there any specific naming conventions for infix functions?
Answer: Infix functions can have any valid function name, but it’s a good practice to choose names that make the code more understandable and maintainable.
What is the difference between a regular function and an infix function in Kotlin?
Answer: Regular functions are called using the conventional function call syntax, while infix functions can be called using the special infix notation, which makes code more natural and expressive. Infix functions are defined with theinfix
keyword and must have one parameter, whereas regular functions have no such restrictions.
What’s the difference between inline and infix function Kotlin?
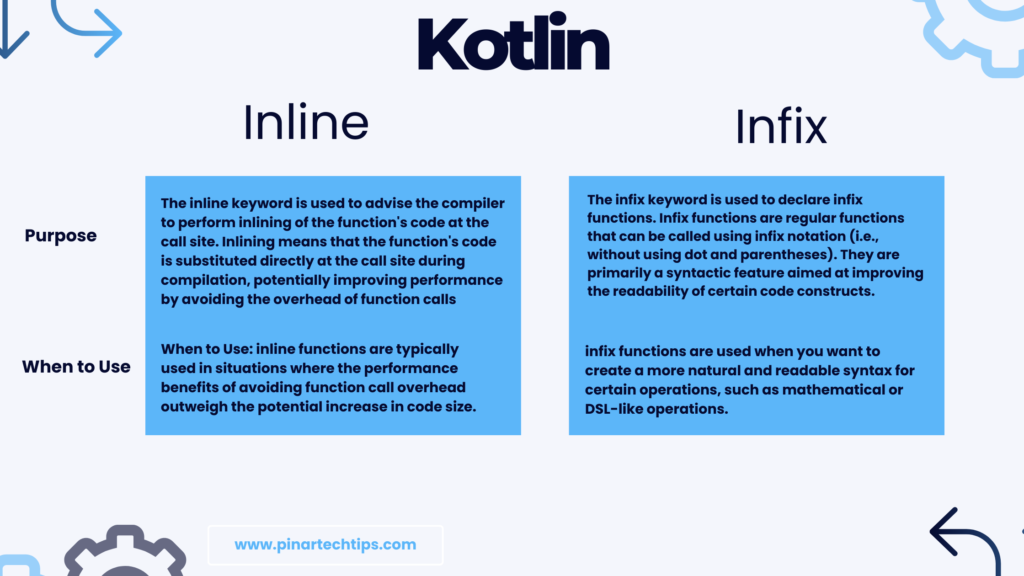
Kotlin Inline Functions
Inline Functions are a feature in Kotlin that allow you to specify that a function should be inlined at the call site. Inlining means that the function’s code is copied directly where it’s called, instead of creating a separate function call, which can improve performance. Here’s an example of an inline function:
inline fun measureTimeMillis(block: () -> Unit): Long {
val startTime = System.currentTimeMillis()
block()
return System.currentTimeMillis() - startTime
}
KotlinIn this example, the measureTimeMillis
function takes a lambda expression as an argument and measures the time it takes to execute the code within that lambda. The inline
keyword indicates that this function should be inlined at the call site.
Kotlin Functions
Advanced Functions | – Higher-Order Functions – Lambda Functions – Extension Functions – Infix Functions- Generic Functions |
Functions | – Simple Functions – Function Parameters – Function Return Types – Function Scope |
Functional Programming Functions | – Immutability and Pure Functions- Function Composition – Functional Operators |
Feel free to follow and join my email list at no cost. Don’t miss out — sign up now!
Please check out my earlier blog post for more insights. Happy reading!
The Innovative Fundamentals of Android App Development in 2023
How to learn Android App Development in 2023 – Full Guide For Beginners using Kotlin Language