
What does Jetpack Compose do?
Jetpack Compose is a declarative UI toolkit for Android, enabling concise UI development through composable functions. It automates rendering and updates based on state, simplifying UI creation and state management.
In the world of app development, creating user interfaces has been traditionally approached in an imperative manner. However, with the advent of technologies like Jetpack Compose, a paradigm shift towards declarative UI development has taken place. In this blog post, we’ll explore the key differences between imperative and declarative UI development, with a special focus on how Jetpack Compose revolutionizes the way we build user interfaces.
Imperative Approach (Traditional Android UI): In traditional Android UI development, you might use Java or Kotlin to create UI elements imperatively, specifying each element’s properties and how they should be displayed.

What is the difference between imperative and declarative approach to UI creation?
he imperative and declarative approaches to UI creation are two contrasting paradigms that developers use to build user interfaces. Here’s a breakdown of the key differences between these two approaches:
Imperative Approach:
- Step-by-Step Instructions: In the imperative approach, developers provide explicit, step-by-step instructions to create and manage each element of the user interface.
- Explicit Updates: Developers are responsible for specifying how the UI should change in response to various events or data changes. This often involves manual updates and modifications to UI elements.
- Detailed Control: This approach offers detailed control over every aspect of the UI. Developers can finely tune how UI elements behave and appear.
- State Management: Imperative UIs often require manual state management, where developers need to keep track of the current state of the application and manually update UI elements to reflect that state.
- Complexity: As the application grows and the UI becomes more complex, the imperative approach can lead to code that is hard to read, maintain, and debug.
Declarative Approach:
- High-Level Descriptions: In the declarative approach, developers describe what the UI should look like based on the current state and conditions. The framework takes care of creating and updating the UI elements accordingly.
- Automatic Updates: Declarative UI frameworks handle updates automatically. When the underlying data changes, the framework calculates the necessary changes to the UI and applies them.
- Abstraction: Developers work at a higher level of abstraction, specifying the desired UI state rather than explicitly detailing how to achieve it. This results in more concise and intuitive code.
- Simplified State Management: Declarative frameworks often provide built-in tools for managing application state, making it easier to handle data changes and updates.
- Code Readability: Declarative code tends to be more readable and expressive. Developers can focus on describing the desired UI structure and behavior rather than managing low-level details.
- Scalability: Declarative UIs are generally more scalable as the application grows. The framework’s automatic reconciliation ensures that updates are efficient and optimized.
- Testing and Preview: Declarative frameworks often provide better tools for testing UI components and real-time previews, aiding development and design iteration.
Notably, Jetpack Compose, a modern UI toolkit by Google, is an example of a declarative approach to UI development for Android applications. It allows developers to describe the UI structure and behavior using composable functions, while the framework takes care of efficiently rendering and updating the UI based on the described state.
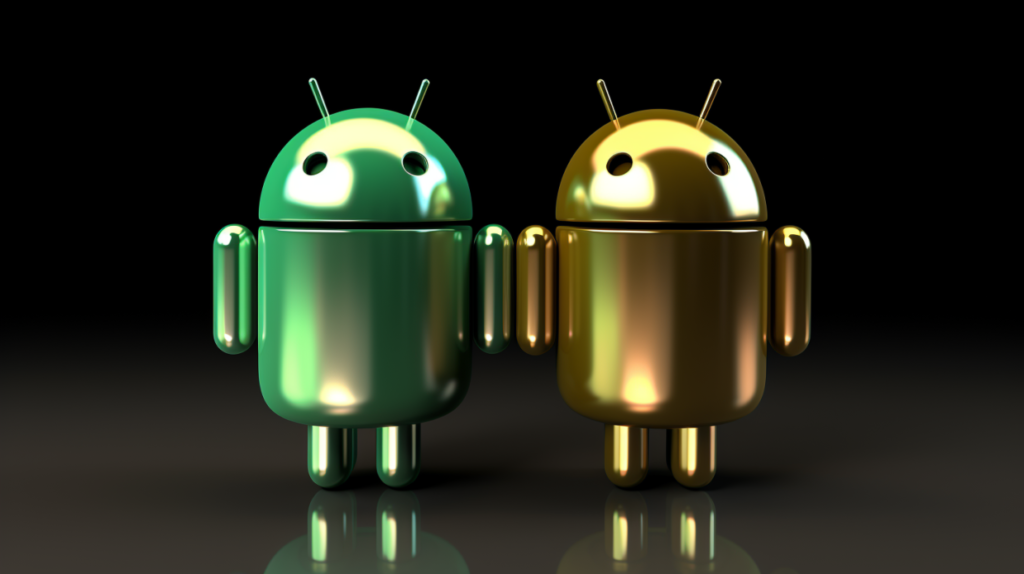
Imperative Approach (Traditional Android UI):
import android.os.Bundle;
import android.widget.Button;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class ImperativeActivity extends AppCompatActivity {
private TextView textView;
private Button button;
private int count = 0;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_imperative);
textView = findViewById(R.id.textView);
button = findViewById(R.id.button);
button.setOnClickListener(view -> {
count++;
textView.setText("Count: " + count);
});
}
}
KotlinIn this imperative example, the UI updates are done imperatively using listeners and manual updates to the TextView.
Declarative Approach using Jetpack Compose:
import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.Column
import androidx.compose.material.Button
import androidx.compose.material.Text
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.ui.tooling.preview.Preview
import com.example.myapplication.ui.theme.MyApplicationTheme
class DeclarativeActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MyApplicationTheme {
DeclarativeScreen()
}
}
}
}
@Composable
fun DeclarativeScreen() {
var count by remember { mutableStateOf(0) }
Column {
Text(text = "Count: $count")
Button(onClick = { count++ }) {
Text(text = "Increment Count")
}
}
}
@Preview(showBackground = true)
@Composable
fun DeclarativePreview() {
MyApplicationTheme {
DeclarativeScreen()
}
}
KotlinIn the declarative example using Jetpack Compose, the UI is described using a set of composable functions. The UI state (count
) is managed using Compose’s state management system, and changes to the state automatically trigger UI updates. There’s no need to manually update UI elements or use listeners.
Key differences:
- In the imperative approach, you manually update UI elements and manage UI state using listeners and callbacks.
- In the declarative approach, you describe the UI hierarchy using composable functions and manage UI state using Compose’s state management, allowing the framework to automatically handle UI updates.
Jetpack Compose’s declarative nature simplifies UI development and eliminates many common sources of UI bugs.
Can I switch from Imperative to Declarative UI Development mid-project?
Yes, transitioning to a declarative approach like Jetpack Compose is feasible, although it might require rewriting parts of the codebase. Start with a smaller component or feature to gain familiarity before committing to a complete shift.
Is Jetpack Compose suitable for all app sizes?
Yes, Jetpack Compose scales effectively for various app sizes. Its declarative nature simplifies the process of managing complex UIs, making it well-suited for both small and large projects.
How does Jetpack Compose enhance developer productivity?
Jetpack Compose’s concise and expressive syntax reduces boilerplate code, resulting in faster development cycles. Its live preview feature further accelerates UI refinement.
Are there any performance considerations with Jetpack Compose?
While Jetpack Compose is designed for optimal performance, developers should be mindful of potential UI component recompositions. Profiling tools can help identify and address performance bottlenecks.
Can I use third-party libraries with Jetpack Compose?
Yes, Jetpack Compose supports integration with existing Android libraries. However, ensure compatibility and follow best practices to prevent conflicts.
What resources are available for learning Jetpack Compose?
The official Jetpack Compose documentation, along with online tutorials and community forums, provide comprehensive resources for learning and mastering the framework.

Feel free to follow and join my email list at no cost. Don’t miss out — sign up now!
Please check out my earlier blog post for more insights. Happy reading!
The Innovative Fundamentals of Android App Development in 2023
How to learn Android App Development in 2023 – Full Guide For Beginners using Kotlin Language
Ultimate Guideline on How to Use ViewModel Design Pattern
What is the Repository Pattern in Android? A Comprehensive Guide
What is ViewModel in Android? A Good Guide for Beginners
The Innovative Fundamentals of Android App Development in 2023
How to Say Goodbye to Activity Lifecycle and Say Hello to Compose Lifecycle ?
How to Monitor/Observe Network Availability for Android Projects
Exploring Sealed Class vs Enum in Kotlin Which is Best for Your Code?
What is the difference between Functional Programming and OOP?