Discover efficient Kotlin control flow and operation practices in this blog, ensuring cleaner, more readable code. Elevate your Kotlin programming skills with essential tips and insights.
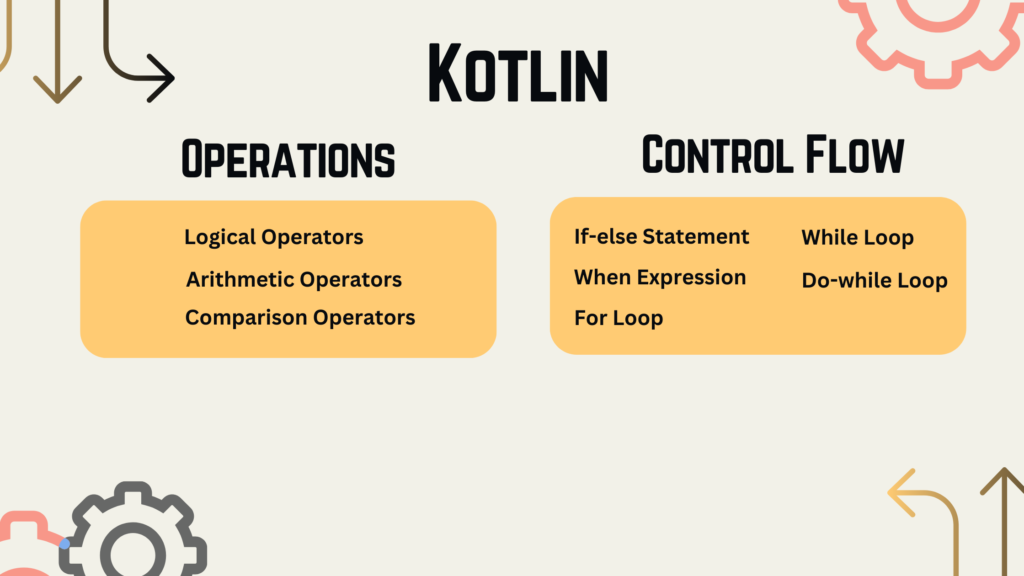
Table of Contents
Kotlin Arithmetic Operators
Arithmetic operators are used to perform mathematical calculations in Kotlin. The primary arithmetic operators are:
- Addition (+): Used to add two numbers together.
- Subtraction (-): Used to subtract the second number from the first.
- Multiplication (*): Used to multiply two numbers.
- Division (/): Used to divide the first number by the second.
- Modulus (%): Used to find the remainder when the first number is divided by the second.
Here’s a simple example of how to use these operators in Kotlin:
val num1 = 10
val num2 = 3
val sum = num1 + num2 // 10 + 3 = 13
val difference = num1 - num2 // 10 - 3 = 7
val product = num1 * num2 // 10 * 3 = 30
val quotient = num1 / num2 // 10 / 3 = 3
val remainder = num1 % num2 // 10 % 3 = 1
KotlinKotlin Comparison Operators
Comparison operators are used to compare values in Kotlin. They help you determine if one value is greater than, less than, equal to, or not equal to another value. The primary comparison operators are:
- Greater than (>): Checks if the left operand is greater than the right.
- Less than (<): Checks if the left operand is less than the right.
- Greater than or equal to (>=): Checks if the left operand is greater than or equal to the right.
- Less than or equal to (<=): Checks if the left operand is less than or equal to the right.
- Equal to (==): Checks if the left operand is equal to the right.
- Not equal to (!=): Checks if the left operand is not equal to the right.
val num1 = 10
val num2 = 3
val isGreaterThan = num1 > num2 // true
val isLessThan = num1 < num2 // false
val isEqual = num1 == num2 // false
val isNotEqual = num1 != num2 // true
KotlinKotlin Logical Operators
Logical operators in Kotlin are essential for controlling the flow of your code and making decisions based on certain conditions. They allow you to work with boolean values (true or false) and determine the logic of your program.
Logical operators are used to combine or modify the results of comparison operations. The primary logical operators are:
- Logical AND (&&): Returns true if both conditions are true.
- Logical OR (||): Returns true if at least one condition is true.
- Logical NOT (!): Returns the opposite of the condition.
Here’s an example of using logical operators:
val isTrue = true
val isFalse = false
val andResult = isTrue && isFalse // false
val orResult = isTrue || isFalse // true
val notResult = !isTrue // false
KotlinProblem: You want to check if a number is both even and greater than 10.
val number = 12
val isEvenAndGreaterThan10 = (number % 2 == 0) && (number > 10)
KotlinProblem: You need to determine if a person is eligible for a discount if they are a student or a senior citizen.
val isStudent = true
val isSeniorCitizen = false
val isEligibleForDiscount = isStudent || isSeniorCitizen
KotlinProblem: You want to verify if a password is invalid (not meeting certain criteria). Solution:
val password = "weak"
val isInvalidPassword = !(password.length >= 8 && password.contains(Regex("[A-Z]")))
KotlinProblem: You need to check if an email address is neither empty nor invalid.
val emailAddress = "user@example.com"
val isEmailValid = !emailAddress.isEmpty() && emailAddress.contains('@') && emailAddress.contains('.')
KotlinProblem: You want to find out if a given year is a leap year (divisible by 4 and not divisible by 100, or divisible by 400).
val year = 2024
val isLeapYear = (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)
KotlinProblem: You want to determine if a user is eligible to access a premium feature if they are both a paying subscriber and their subscription is active.
val isPayingSubscriber = true
val isSubscriptionActive = true
val canAccessPremiumFeature = isPayingSubscriber && isSubscriptionActive
KotlinProblem: You are developing a ride-sharing app and need to determine if a driver can accept a ride request. The driver should be logged in, have a valid license, and have their vehicle’s insurance up to date.
val isDriverLoggedIn = true
val hasValidLicense = true
val isInsuranceUpToDate = true
val canAcceptRideRequest = isDriverLoggedIn && hasValidLicense && isInsuranceUpToDate
KotlinOperator Precedence
Operator precedence determines the order in which operations are evaluated in an expression. Some operators are evaluated before others. In Kotlin, the order of precedence is as follows (from highest to lowest):
- Arithmetic operators (*, /, %)
- Addition and subtraction operators (+, -)
- Comparison operators (>, <, >=, <=, ==, !=)
- Logical NOT (!)
- Logical AND (&&)
- Logical OR (||)
val result = (num1 + num2) * (num1 - num2)
KotlinThis expression first adds num1
and num2
, then subtracts num2
from the result.
Problem: You need to calculate the final price of an item with a discount and tax applied. The discount should be applied first, followed by the tax.
val originalPrice = 100
val discountPercentage = 20
val taxPercentage = 8
val finalPrice = (originalPrice - (originalPrice * discountPercentage / 100)) * (1 + taxPercentage / 100)
KotlinProblem: You want to calculate the total cost of items in a shopping cart, including shipping costs. Shipping costs should only apply if the total cart value is less than a certain threshold.
val cartTotal = 200
val shippingThreshold = 100
val shippingCost = if (cartTotal < shippingThreshold) 10 else 0
val totalCost = cartTotal + shippingCost
KotlinProblem: You are building a time tracking app, and you need to calculate the total hours and minutes worked based on user input for start and end times.
val startTimeHours = 9
val startTimeMinutes = 30
val endTimeHours = 17
val endTimeMinutes = 45
val totalHours = endTimeHours - startTimeHours + (endTimeMinutes - startTimeMinutes) / 60
val totalMinutes = (endTimeMinutes - startTimeMinutes) % 60
KotlinProblem: You want to determine a user’s age from their birthdate and show different content based on whether they are a child, teenager, or an adult.
val birthYear = 2000
val currentYear = 2023
val age = currentYear - birthYear
val userCategory = when {
age < 13 -> "Child"
age < 18 -> "Teenager"
else -> "Adult"
}
KotlinKotlin Control Flow
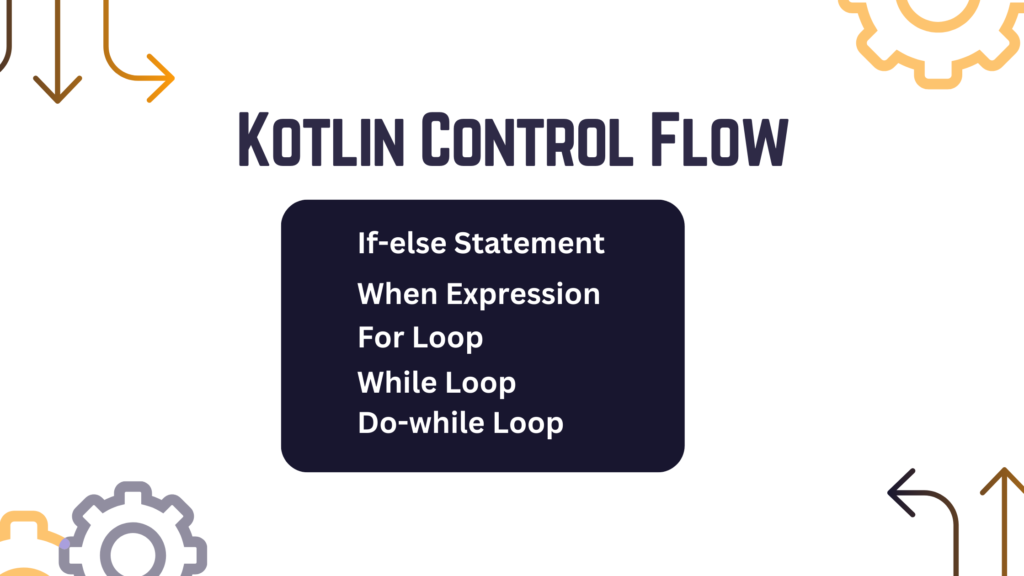
if-else Statement:
- The
if
statement is used for conditional execution. It can be followed by an optionalelse
block.
val x = 10
if (x > 0) {
println("Positive")
} else if (x < 0) {
println("Negative")
} else {
println("Zero")
}
Kotlinwhen Expression:
- The
when
expression is Kotlin’s replacement for the traditionalswitch
statement. It allows for concise and powerful branching based on multiple conditions. - The
when
expression in Kotlin is a powerful and concise replacement for the traditionalswitch
statement found in some other programming languages. It allows you to express complex conditional logic in a more readable and flexible way.
val day = 3
when (day) {
1 -> println("Monday")
2 -> println("Tuesday")
in 3..5 -> println("Wednesday to Friday")
else -> println("Weekend")
}
Kotlinfor Loop:
- The
for
loop is used for iterating over a range, collection, or any other iterable
for (i in 1..5) {
println(i)
}
Kotlinwhile Loop:
- The
while
loop executes a block of code repeatedly as long as a given condition is true.
var i = 1
while (i <= 5) {
println(i)
i++
}
Kotlindo-while Loop:
- The
do-while
loop is similar to thewhile
loop, but it guarantees that the block of code is executed at least once before checking the loop condition.
var i = 1
do {
println(i)
i++
} while (i <= 5)
Kotlin- If-Else Statements:
- Use Case: Condition with two possible outcomes.Scenario: Weather app checking if temperature is above a threshold.
- When Expression:
- Use Case: Multiple conditions with different actions.Scenario: Music player app handling playback states.
- For Loops:
- Use Case: Iterating over a collection or range.Scenario: News app displaying articles in a RecyclerView.
- While Loops:
- Use Case: Repeatedly executing code while a condition is true.Scenario: Game app updating state and rendering frames.
- do-while Loop:
- Use Case: when you want to ensure that a certain block of code is executed at least once, regardless of the initial condition.
When choosing a control flow structure, consider readability, maintainability, and the specific requirements of your application. Kotlin’s concise syntax and expressiveness make it easy to write clean and efficient code for various scenarios in Android development.
Question: Sum of Even Numbers
- Write a Kotlin function that takes an integer
n
as a parameter and calculates the sum of all even numbers from 1 ton
. Use a loop for this task
fun sumOfEvenNumbers(n: Int): Int {
var sum = 0
for (i in 2..n step 2) {
sum += i
}
return sum
}
// Example usage:
val result = sumOfEvenNumbers(10)
println(result)
// Output: 30
KotlinThis Kotlin function calculates the sum of all even numbers from 2 to n
. It uses a for
loop to iterate through even numbers (skipping odd numbers) and accumulates their sum. The step 2
in the loop ensures that only even numbers are considered.
Kotlin Control Flow and Operations Best Practices
In Android development, one common use of a while
loop is for iterating through a collection of data retrieved from a database or an API. Let’s consider an example where we have a list of user data retrieved from a hypothetical API, and we want to perform some operation for each user until a certain condition is met.
class User(val id: Int, val name: String)
// Example usage in an Android ViewModel
class UserViewModel : ViewModel() {
private val userRepository = UserRepository() // Assume UserRepository handles data retrieval
fun performOperationForUsers() {
val userList = userRepository.getUserList()
var index = 0
while (index < userList.size && !userList[index].name.equals("John", ignoreCase = true)) {
// Perform some operation for each user until we find a user named "John"
val currentUser = userList[index]
performOperation(currentUser)
// Move to the next user in the list
index++
}
}
private fun performOperation(user: User) {
// Some operation to be performed for each user
// e.g., Display user information, update UI, etc.
Log.d("UserOperation", "Performing operation for user: ${user.name}")
}
}
KotlinIn this example:
- The
User
class represents a user with an ID and a name. - The
UserViewModel
is an Android ViewModel responsible for interacting with user data. - The
performOperationForUsers
function retrieves a list of users from theUserRepository
and performs some operation for each user until it finds a user named “John” or reaches the end of the list. - The
performOperation
function represents the operation to be performed for each user. In a real application, this could involve updating the UI, making network requests, or other tasks.
This example demonstrates the use of a while
loop to iterate through a list of users until a certain condition is met. The loop is terminated when it encounters a user named “John” or when it reaches the end of the list.
Keep in mind that in Android development, asynchronous operations like database queries or network requests are typically performed on background threads or using coroutines. The example above simplifies the scenario for demonstration purposes.
Using the when
expression in an Android application
fun getCategoryName(categoryId: Int): String {
return when (categoryId) {
1 -> "Electronics"
2 -> "Clothing"
3 -> "Books"
4, 5 -> "Other Categories" // Multiple values can be grouped together
in 6..10 -> "Custom Range" // Check if the value is in a range
else -> "Unknown Category"
}
}
fun main() {
val category1 = getCategoryName(2)
val category2 = getCategoryName(7)
println("Category 1: $category1") // Output: Clothing
println("Category 2: $category2") // Output: Custom Range
}
KotlinIn this example:
- The
getCategoryName
function takes acategoryId
as a parameter and uses awhen
expression to determine the corresponding category name. - It checks the value of
categoryId
against specific values, ranges, or conditions. If a match is found, the corresponding category name is returned. If none of the conditions match, theelse
branch is executed. - The
main
function demonstrates callinggetCategoryName
with different values and printing the results.
The when
expression is especially useful for replacing long chains of if-else if
statements, making the code more concise and readable. It supports various forms of pattern matching, including value checks, range checks, and more complex conditions.

Feel free to follow and join my email list at no cost. Don’t miss out — sign up now!
Please check out my earlier blog post for more insights. Happy reading!
The Innovative Fundamentals of Android App Development in 2023
How to learn Android App Development in 2023 – Full Guide For Beginners using Kotlin Language
Ultimate Guideline on How to Use ViewModel Design Pattern
What is the Repository Pattern in Android? A Comprehensive Guide
What is ViewModel in Android? A Good Guide for Beginners
The Innovative Fundamentals of Android App Development in 2023
How to Say Goodbye to Activity Lifecycle and Say Hello to Compose Lifecycle ?
How to Monitor/Observe Network Availability for Android Projects
Exploring Sealed Class vs Enum in Kotlin Which is Best for Your Code?